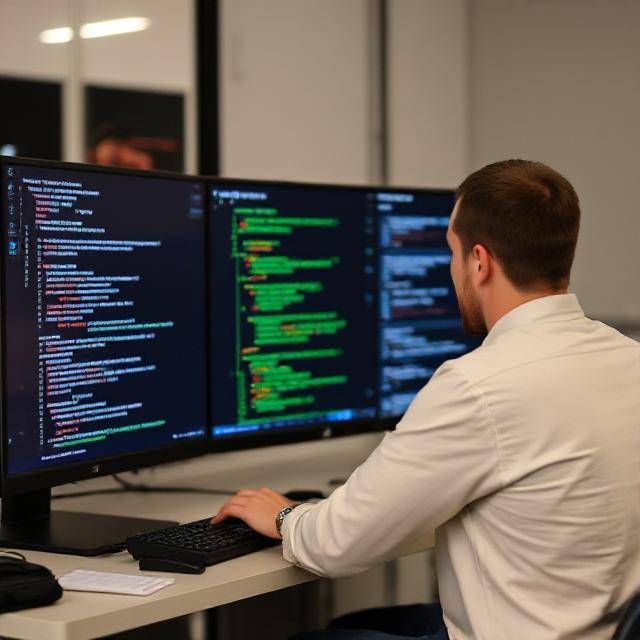
Web scraping is a powerful technique for extracting data from websites. In this beginner’s guide, you will learn how to get started with web scraping using Python, specifically focusing on Beautiful Soup and the Requests library.
What is Web Scraping?
Web scraping is a technique used to extract data from websites. It involves using specialized software to navigate a website, extract specific data, and save it to a local file or database. Web scraping is useful for data analysis, research, and various tasks such as extracting contact information from websites, tracking prices, and monitoring social media trends.
Tools and Libraries Needed
For web scraping with Python, you’ll need the following libraries:
- Requests: A library for sending HTTP requests to websites.
- Beautiful Soup: A library for parsing HTML and XML documents.
Getting Started with Beautiful Soup and Requests
Step 1: Install the Required Libraries
To start web scraping with Python, you need to install the required libraries. Open your terminal or command prompt and install the libraries using pip:
Copypip install requests beautifulsoup4
Step 2: Send an HTTP Request to a Website
First, you need to send an HTTP request to a website to obtain its HTML content. You can use the requests
library for this purpose.
Copyimport requests # Send an HTTP GET request to the website url = "http://www.example.com" response = requests.get(url) # Check if the request was successful if response.status_code == 200: print("Request successful!") else: print("Request failed!")
Step 3: Parse the HTML Content with Beautiful Soup
Next, you need to parse the HTML content of the website using the Beautiful Soup
library.
Copyfrom bs4 import BeautifulSoup # Parse the HTML content soup = BeautifulSoup(response.content, "html.parser") # Print the parsed HTML content print(soup.prettify())
Step 4: Extract Data from the Website
Now, you can extract specific data from the website using Beautiful Soup.
Copy# Extract all the links from the website links = soup.find_all("a") # Print the extracted links for link in links: print(link.get("href"))
Example Code
Here’s the complete example code combining the above steps:
Copyimport requests from bs4 import BeautifulSoup # Send an HTTP GET request to the website url = "http://www.example.com" response = requests.get(url) # Check if the request was successful if response.status_code == 200: print("Request successful!") else: print("Request failed!") # Parse the HTML content soup = BeautifulSoup(response.content, "html.parser") # Print the parsed HTML content print(soup.prettify()) # Extract all the links from the website links = soup.find_all("a") # Print the extracted links for link in links: print(link.get("href"))
That’s it! You’ve successfully started web scraping with Python using Beautiful Soup and the Requests library.
Tips and Best Practices
- Always check the website’s terms of use to ensure that web scraping is allowed.
- Use a user-agent header to identify your scraper and avoid being blocked.
- Respect website bandwidth limitations and avoid overwhelming the server with requests.
- Use Beautiful Soup’s
find
andfind_all
methods to extract specific data, and consider using CSS selectors for more complex queries.
Common Issues and Solutions
- Request failed: Check the website’s status code and ensure that the request was successful.
- Beautiful Soup parsing failed: Try using a different parser (e.g.,
html5lib
orlxml
) or check the HTML content for errors. - Data extraction failed: Use Beautiful Soup’s
find
andfind_all
methods to extract specific data, and consider using CSS selectors for more complex queries.
That’s it! You’re now ready to start web scraping with Python using Beautiful Soup and the Requests library.
Залишити відповідь