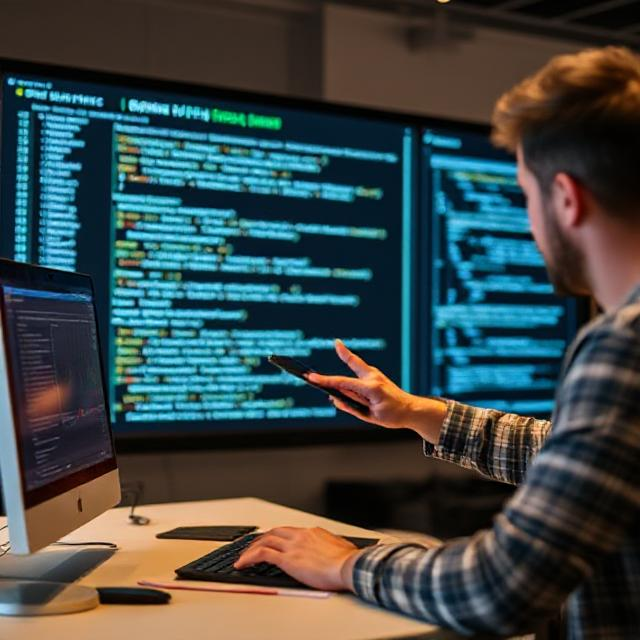
Here’s a breakdown of best practices for writing modular and maintainable code, covering key principles, techniques, and tools:
I. Core Principles
- Modularity:
- Definition: Breaking down a program into independent, self-contained modules (also often called “components,” “units,” or “classes”) with well-defined responsibilities.
- Benefits:
- Reusability: Modules can be reused in different parts of the application or even in other projects.
- Testability: Individual modules can be tested in isolation, making it easier to identify and fix bugs.
- Maintainability: Changes to one module are less likely to affect other parts of the code.
- Collaboration: Makes it easier for multiple developers to work on the same project.
- Readability: Increases the legibility of the code.
- Abstraction:
- Definition: Hiding complex implementation details and exposing only essential information.
- Benefits:
- Simplifies the interface of a module.
- Allows the internal implementation to be changed without affecting the code that uses the module (encapsulation).
- Reduces complexity.
- Provides a clean API.
- Encapsulation:
- Definition: Bundling data (attributes/properties) and methods (functions/operations) that operate on that data within a module and controlling access to that data (using access modifiers like
private
,protected
,public
). - Benefits:
- Protects data from being accessed or modified in ways that could lead to errors.
- Allows for internal implementation changes without affecting external code.
- Improves data integrity.
- Definition: Bundling data (attributes/properties) and methods (functions/operations) that operate on that data within a module and controlling access to that data (using access modifiers like
- Single Responsibility Principle (SRP):
- Definition: A module should have only one reason to change. In other words, a module should do one thing and do it well.
- Benefits:
- Reduces complexity.
- Makes modules easier to understand, test, and maintain.
- Minimizes the impact of changes.
- Don’t Repeat Yourself (DRY):
- Definition: Avoid duplicating code. If you find yourself writing the same code in multiple places, abstract it into a function or module.
- Benefits:
- Reduces code duplication.
- Simplifies maintenance (one change in one place).
- Improves consistency.
II. Techniques & Tools
- Choosing Appropriate Module Size:
- Too Small: Can lead to excessive inter-module dependencies and increased complexity.
- Too Large: Can be difficult to understand, test, and reuse.
- Guideline: Modules should be large enough to be meaningful but small enough to have a clear, focused purpose.
- Meaningful Naming:
- Variables: Names that accurately describe the data they hold.
- Functions/Methods: Names that clearly indicate what the function does.
- Classes/Modules: Names that reflect the responsibilities of the module.
- Consistency: Use a consistent naming convention throughout the codebase (e.g., camelCase, snake_case, PascalCase).
- Clear Documentation:
- Comments: Use comments to explain the purpose of code blocks, functions, and classes, especially for complex logic.
- Docstrings/Documentation Generators: Write comprehensive documentation for modules, functions, and classes, including input parameters, return values, and usage examples.
- Code Reviews:
- Process: Peer review of code is a crucial practice.
- Benefits:
- Helps catch errors and enforce coding standards.
- Promotes knowledge sharing.
- Improves code quality.
- Version Control (Git):
- Use Git: Essential for tracking code changes, collaborating with others, and reverting to previous versions.
- Dependency Management:
- Tools: Use dependency management tools (e.g.,
npm
,pip
,maven
,bundler
) to manage external libraries and their versions. - Avoid Circular Dependencies: Circular dependencies can create complex, hard-to-maintain code.
- Tools: Use dependency management tools (e.g.,
- Testing:
- Unit Tests: Test individual modules in isolation.
- Integration Tests: Test the interaction between modules.
- Test-Driven Development (TDD): Write tests before you write the code.
- Refactoring:
- Definition: Improving the internal structure of code without changing its external behavior.
- Techniques:
- Extract Method: Extract code blocks into separate functions.
- Extract Class: Create a new class to encapsulate related functionality.
- Rename: Rename variables and functions for clarity.
- Move Method/Field: Move methods and fields to the appropriate classes.
- Design Patterns:
- Use Design Patterns: Employ established design patterns (e.g., Factory, Strategy, Observer) to solve common software design problems.
- Code Style Guides/Linters:
- Follow a Code Style Guide: Adhere to a consistent code style (e.g., PEP 8 for Python, Airbnb JavaScript Style Guide) to improve readability.
- Linters: Use linters (e.g.,
pylint
for Python,ESLint
for JavaScript) to automatically check your code for style violations and potential errors.
III. Code Structure and Organization
- Directory Structure:
- Organize your code into a logical directory structure that reflects the modularity of your application.
- Group related modules together.
- Use clear directory names.
- Separation of Concerns:
- Segregate code based on its function or role (e.g., UI code, business logic, data access).
IV. Example (Python-ish)
Copy# Bad (Monolithic) def calculate_rectangle_area(length, width, unit): """Calculates the area of a rectangle and formats the result.""" area = length * width formatted_area = f"{area} {unit}²" print(f"The area is: {formatted_area}") # Mixed functionality return formatted_area # Good (Modular) class Rectangle: """Represents a rectangle.""" def __init__(self, length, width): self.length = length self.width = width def calculate_area(self): """Calculates the area.""" return self.length * self.width class AreaFormatter: """Formats area values.""" @staticmethod def format_area(area, unit): """Formats the area with the given unit.""" return f"{area} {unit}²" # Usage rectangle = Rectangle(5, 10) area = rectangle.calculate_area() formatted_area = AreaFormatter.format_area(area, "cm") print(f"The area is: {formatted_area}")
V. Summary
Writing modular and maintainable code requires discipline, careful planning, and attention to detail. By following these best practices, you can create code that is easier to understand, test, debug, and modify, leading to more robust and sustainable software. Consistent application of these principles over time leads to a high-quality codebase.
Залишити відповідь