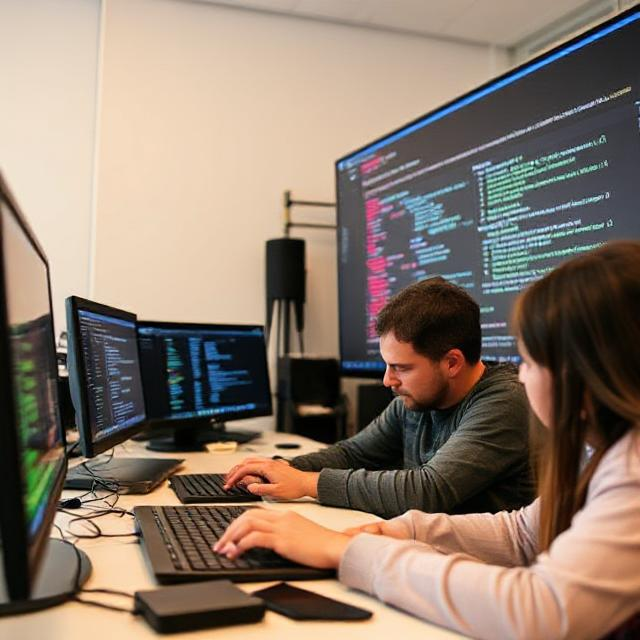
Functional programming is a paradigm in which programming is performed using pure functions, immutability, recursion, and the avoidance of changing-state and mutable data. This approach focuses on evaluating expressions to produce new data, rather than changing existing data or maintaining the state.
Benefits of Functional Programming
Some of the key benefits of functional programming include:
- Modularity: Functional programming encourages modularity, making it easier to write, test, and maintain code. This modularity also makes it easier to compose functions together to solve complex problems.
- Predictability: Because functional programming code is typically side-effect-free, the outcome of executing the code is easier to predict. This predictability reduces debugging and troubleshooting times.
- Readability: Functions and expressions are typically smaller and easier to understand than imperative code, making it easier for new developers to join the project and understand the codebase.
- Parallelism: Functional programming lends itself well to parallelization, making it ideal for concurrent or distributed computing.
- Less Bug-Prone: Immutable data structures and the absence of changing-state reduce the likelihood of bugs caused by data corruption or unintended side effects.
Challenges of Functional Programming
However, there are also several challenges associated with functional programming:
- Steeper Learning Curve: Functional programming concepts can be difficult to grasp for developers who are accustomed to imperative programming.
- Performance Implications: Some functional programming techniques, like recursion and immutable data structures, can have significant performance implications.
- Memory Efficiency: Immutable data structures often require more memory than their mutable counterparts.
- Debugging: The predictability of functional programming can make it more challenging to debug, as the code’s behavior is determined by the function’s inputs and the expression’s outputs.
- Error Handling: Functional programming’s emphasis on pure functions and immutability can make it more difficult to handle and propagate errors.
Functional Programming in Real-World Applications
Here are a few real-world applications and industries where functional programming has been successfully applied:
- Artificial Intelligence (AI): Functional programming has been employed in AI for tasks such as machine learning, natural language processing, and computer vision.
- Data Analysis and Science: Functional programming has been utilized in data analysis and science for tasks like data transformation, aggregation, and statistical analysis.
- Web Development: Functional programming has been applied in web development for building scalable, concurrent, and maintainable web applications.
- Machine Learning: Functional programming has been used in machine learning for tasks such as data preprocessing, feature engineering, and model evaluation.
Popular Functional Programming Languages and Frameworks
Here are some popular functional programming languages and frameworks:
- Haskell: A purely functional programming language with strong, static typing and garbage collection.
- Lisp: A family of programming languages with a long history, known for their functional programming paradigm and macro system.
- Scala: A multi-paradigm language that supports both object-oriented and functional programming styles.
- Clojure: A general-purpose programming language with a focus on immutability, recursion, and immutable data structures.
- Elixir: A dynamic language with a focus on concurrency, parallel processing, and distributed programming.
Best Practices for Applying Functional Programming
To successfully apply functional programming concepts to real-world applications:
- Follow the “Pure” Principle: Ensure that your functions are “pure” (i.e., their outputs depend only on their inputs and not on external variables or the global state).
- Use Immutability: Prefer immutable data structures over mutable ones to make your code more predictable and less error-prone.
- Emphasize Recursion: Recursion can be a powerful tool in functional programming, making it easier to solve complex problems recursively.
- Focus on Functions as First-Class Citizens: Treat functions as first-class citizens in your code, using them in function composition and higher-order functions.
- Learn and Practice: Learn from existing functional programming libraries, and practice your skills in solving real-world problems.
Залишити відповідь